Node.js TypeScript Best Repository Setup for Local Dev Environment
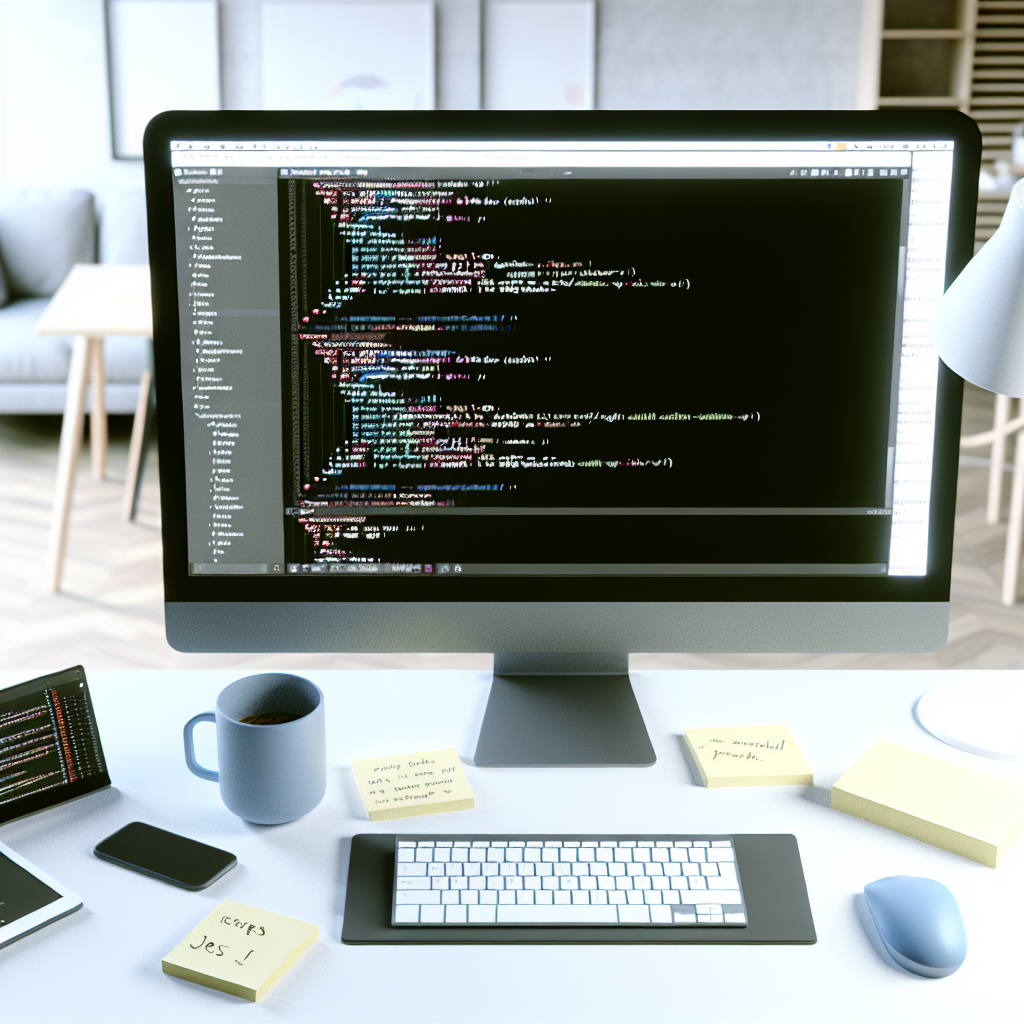
Table of Contents
Introduction
Setting up a Node.js repository with TypeScript for local development can significantly boost your productivity and maintainability of your codebase. This article will guide you through the best practices for creating an efficient and scalable development environment. From initial setup to continuous integration, we’ll cover everything you need to know to get started.
Why Choose TypeScript?
TypeScript is a superset of JavaScript that adds static typing to the language. This helps in catching errors early during the development phase rather than at runtime. The additional type information can also improve code readability and maintainability. By using TypeScript in your Node.js projects, you can leverage features like interfaces, enums, and type aliases to create more robust and self-documenting code.
Initial Setup
The first step in setting up your repository is initializing a new Node.js project. You can do this by running npm init
in your project directory. This command will guide you through creating a package.json
file, which will hold metadata about your project and its dependencies. Once the project is initialized, you should install TypeScript and other necessary packages by running npm install typescript ts-node @types/node --save-dev
.
Configuring TypeScript
After installing TypeScript, the next step is to configure it. Create a tsconfig.json
file in the root of your project. This file will contain the TypeScript compiler options. A basic configuration might look like this:
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true
}
}
This setup ensures that your TypeScript code is compiled down to a version of JavaScript that Node.js can execute.
Organizing Your Project Structure
A well-organized project structure is crucial for maintainability. A common structure for a Node.js TypeScript project might look like this:
my-project/
|-- src/
| |-- index.ts
| |-- controllers/
| |-- models/
| |-- services/
|-- dist/
|-- node_modules/
|-- package.json
|-- tsconfig.json
|-- .gitignore
This structure separates your source code from the compiled JavaScript files, which will reside in the dist
directory.
Setting Up Scripts
To streamline your development workflow, you should set up npm scripts in your package.json
. These scripts can automate common tasks like building and running your project. Here’s an example of how you might configure these scripts:
"scripts": {
"build": "tsc",
"start": "node dist/index.js",
"dev": "ts-node src/index.ts"
}
The build
script runs the TypeScript compiler, the start
script runs your compiled JavaScript code, and the dev
script allows you to run your TypeScript code directly for development purposes.
Using Linting and Formatting Tools
Linting and formatting tools help maintain code quality and consistency. ESLint is a popular linting tool for JavaScript and TypeScript. You can install it by running npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
. After installation, create a .eslintrc.json
file for your ESLint configuration. Prettier is another tool that helps in automatically formatting your code. Install it by running npm install prettier --save-dev
and create a .prettierrc
file for the configuration.
Setting Up Testing
Testing is an integral part of modern development. Jest is a popular testing framework that works well with TypeScript. You can install it by running npm install jest ts-jest @types/jest --save-dev
. After installation, create a jest.config.js
file to configure Jest for TypeScript. This setup ensures that your code is thoroughly tested before deployment.
Continuous Integration
Setting up continuous integration (CI) helps in automating the testing and deployment process. GitHub Actions is a popular choice for CI. Create a .github/workflows/ci.yml
file to define your CI pipeline. This file will contain instructions for running tests and building your project whenever you push changes to your repository.
Conclusion
Setting up a Node.js TypeScript repository for local development involves several steps, from initial setup to configuring CI. By following these best practices, you can create a robust and maintainable development environment. This will not only make your development process more efficient but also ensure the long-term success of your project.